How to use Firebase Storage and Firebase Database with React?
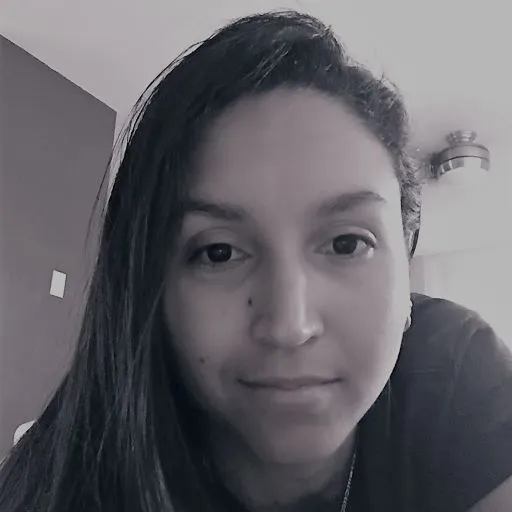
By:
Denisse Abreu
April 25, 2023 8pm ET
Eng/Spa 8-min
Hello 👋🏼, let's continue with the Firebase series by learning how to
work with the Storage and the Database. We'll create a simple shopping cart to sell cups with
an admin form to illustrate how to upload images and retrieve them from the database
with their corresponding product. We'll continue to work with React, TypeScript, and
Tailwind CSS.
Let's start!
Initialize Firebase Database and Storage for Web Apps.
Go to the Build section on the left side of the screen, provision the Storage and the Database set it all in testing mode. Then, go to the rules tab and add the following rules. Follow Get Started with Cloud Storage on Web and Get Started with Cloud Firestore if you need extra help.
- Storage Rules.
storage rulesrules_version = '2'; service firebase.storage { match /b/{bucket}/o { match /{images}/{imageName} { allow read; allow write: if // Public access for development purposes. // Allow 5 MB image or less. request.resource.size < 5 * 1024 * 1024 && request.resource.contentType.matches('image/.*'); } } }
- Firestore Database Rules.
firestore database rulesrules_version = '2'; service cloud.firestore { match /databases/{database}/documents { match /{store}/{productId} { // Public access for development purposes. allow read, write; } } }
- Create a React Typescript project with Vite and install Firebase.
Git Bashnpm create vite@latest file-upload-tutorial -- --template react-ts && cd file-upload-tutorial && npm install firebase
How to upload images to the Firebase storage?
After setting up the React project, we'll code the admin form
and the Firebase file. First, create the utils folder, and inside
create firebase.ts
.
Git Bash
cd src
mkdir utils && cd utils
touch firebase.ts
-
Insert the following code in the
firebase.ts
file. Don't forget to add your Firebase config keys!
src/utils/firebase.ts
-
Create the components folder and inside create
adminForm.tsx
. For the CSS styling, I'm using Tailwind CSS.
Git Bash
cd src
mkdir components && cd components
touch adminForm.tsx
As you can notice the file upload input is separated from the other
input elements because it targets a different object called
FileList
. Extract from
the FileList the name and the complete object. Send them as attributes to the
uploadProduct()
function.
src/components/adminForm.tsx
-
Bring the form to the
App.tsx
src/App.tsx
After uploading your first image, go to Firebase and check that the images folder is in the storage and the database has the product with the image URL.
How to get the shopping cart products from the Firebase database?
Now that you can upload images and data, it's time to retrieve it.
Go to the firebase.ts
file and add the
following code. Notice that we're importing DocumentData[]
type from Firebase.
src/utils/firebase.ts
-
Create two more components
productCards.tsx
andproductCardInfo.tsx
.
Git Bashcd components touch productCards.tsx && touch productCardInfo.tsx
Copy the following code into the productCards.tsx
;
this component is in charge of fetching the database and
showing a grid of products.
src/components/productCards.tsx
Our second component is the productCardInfo.tsx
;
this component displays the inside of the card with
the product information.
src/components/productCardInfo.tsx
-
Bring the two components to the
App.tsx
, refresh the browser, and you should see the products!
src/App.tsx
Add the React Context for dynamic product fetching.
Right now, the products appear on the screen when you refresh
the web page; this is totally fine for a shopping store; however,
if you want to display the products dynamically when hitting the
submit button, you need to create a Context. Every time the
getProducts()
function is called
the Context will instruct the product card component to re-render
showing the latest product you added to the database.
-
First, create a
context
folder, and inside createproducts-context.tsx
.
Git Bash
cd src
mkdir context && cd context
touch products-context.tsx
-
Copy the following code inside the
products-context.tsx
src/context/product-context.tsx
-
Add the Context to the
main.tsx
src/main.tsx
-
Called the
setProducts()
function in the Admin form.
src/components/adminForm.tsx
Delete the product state and the useEffect()
;
now you can display the products dynamically
using the Context, see you next time!
src/components/productCards.tsx