Connect Stripe to a React TypeScript Ecommerce.
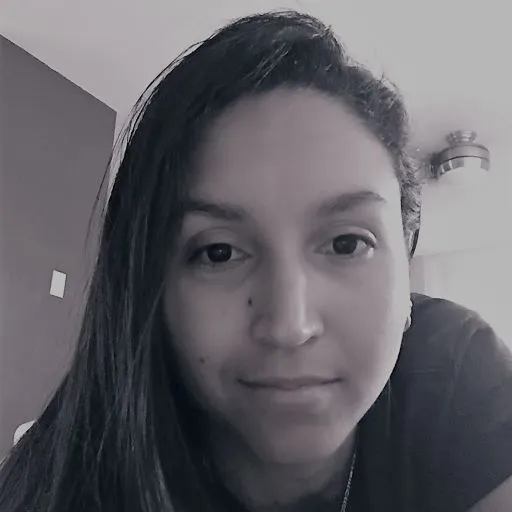
By:
Denisse Abreu
August 16, 2022 8pm ET
Eng/Spa 7-min
Hello 👋🏼, In this tutorial, I will teach you how to connect Stripe to a React TypeScript Shopping Store. For this integration, you will need to be comfortable dealing with React and TypeScript and, of course, an Ecommerce website. Our working environment is React TypeScript, React Router Dom v6, and Express js as a backend manager. If you don't have an Ecommerce website, you can fork my Mini Monster Store in my Github repository.
Payment Workflow
Set Up your Stripe Account.
To get started with Stripe, you need to open a Stripe account;
After opening your account, go to the developer's section and access your API keys.
In the developer's section, you will find two keys: publishable key y secret key; copy the secret key in your server .env file.
.env
Stripe dashboard
Connect the Express js Server to your React App.
First, start by installing the Stripe library; I will use Express js as a server, but you can use your preferred server framework. Consult the Stripe guide for the different server languages, you can use.
Install through npm
npm install stripe --save
Paste the following code into your index.ts in the root of the Express js server. If you don't know how to set up an Express js server with TypeScript, go to my tutorial Create a React and Express js project with TypeScript and follow the first part of how to set up Express js TypeScript.
index.ts
-
When your server is ready, open your terminal and run:
npm run dev
and start listening to the client-side API requests.
Set Up the Stripe Integration in your React App.
- Create the .env file in the root of the Mini Monster Store and copy your publishable key from Stripe.
.env
- Open your terminal and Install the React Stripe.js library.
Install through npm
npm install --save @stripe/react-stripe-js @stripe/stripe-js
- Go to the Utils folder and create the Stripe folder; inside this folder create payment-intent-utils.ts; this file will be in charge of making the API call to the server.
Git Bashcd utils mkdir stripe cd stripe touch payment-intent-utils.ts
- Paste the following code inside the payment-intent-utils.ts file; It contains the Fetch() call to the server with the URL and the total amount multiplied by 100. Remember, Stripe will not accept decimal numbers.
utils/stripe/payment-intent-utils.ts
Go to the subtotal card, import the payment intent function from the utils folder, and copy the try and catch block inside the checkout constant. When the customer clicks the subtotal button, a call to the server will be fired asking for the client secret key. If the call is successful, your customer will be redirected to the checkout route with the client secret as a parameter. This integration uses React Router for easy moving between the shopping store pages.
components/subtotal-card/subtotal-card.tsx
Open the checkout route and import from the react-router-dom useLocation() function. This function will be in charge of getting the client secret that we send from the subtotal card; pass this client secret as a prop to the checkout card.
routes/checkout.tsx
Go to the components folder and create the payment-form folder; inside of that folder, create the payment-form.tsx file. This file will be in charge of showing the Stripe Payment Element to the customer. There are two form inputs in the payment form to showcase how to collect data from the customer; If you don't know how to make reusable input components go to the third part of my tutorial Create a React and Express js project with TypeScript.
Git Bashcd components mkdir payment-form cd payment-form touch payment-form.tsx
components/payment-form/payment-form.tsx
Now comes the heavy part; Go to the checkout card and import Elements, loadStripe, and the payment form; get the client secret from the checkout route, and use it to show your customer the Stripe Payment Element. Customize the Stripe Appearance to match your UI.
components/checkout-card/checkout-card.tsx
After sending the form, we need to check the payment status from Stripe. Create the payment-status.ts file and put it inside the Stripe folder under utils. This code will run a series of switch cases to set the corresponding error message and additional logic like clearing the cart on a successful transaction or going back if there is an error.
Git Bashcd utils cd stripe touch payment-status.ts
utils/stripe/payment-status.ts
The last part, glad you made it here! Go to the thankyou route and import the payment status function and put it inside the Stripe Elements component; I made a simple alert box to show the customer the payment status; you can make something different here, see you next time!
routes/thankyou.tsx