How to Connect Stripe to a Vue 2 Online Shopping Store?
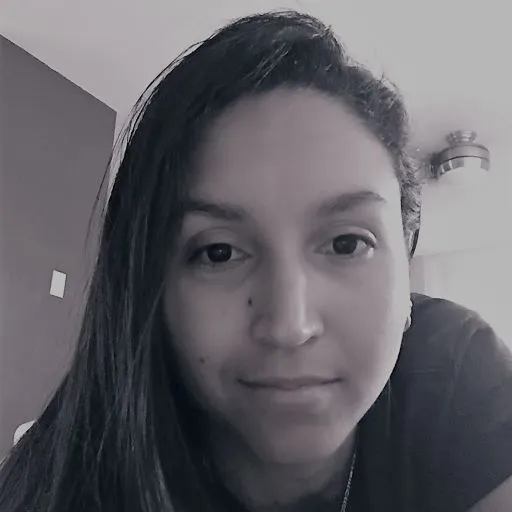
By:
Denisse Abreu
Mar 2, 2022 12pm ET
Eng/Spa 6-min
Hi 👋, in this guide, I will teach you how to connect Stripe to Vue 2. This integration is for shopping carts only, not subscriptions. If you want to check the backend first do it here: How to Connect Stripe to Django Rest Framework? We'll assume you already know how to work in a Vue js environment. If you don't have a project, you can copy mine here: Django Shopping Store.
How does Stripe work?
Stripe recommends once you know the transaction subtotal you should send it to your server.
In your server, calculate the total using the product id. Always make calculations on the server;
it will stop people from messing up with your prices. Once you have the calculations send them to Stripe using the paymentIntent.create()
function.
At Stripe they will receive the transaction, mark it as incomplete and return the payment intent with the client secret.
This payment intent object with the client secret is important; send it to the frontend (Vue js) and save it in the state.
Later, we will use the client secret to mount the card element so your customer can complete the transaction. Remember, in the end, to clear the state and the Stripe client secret.
1. Set Up the Stripe Environment.
To get started with Stripe, you need to open a Stripe account;
After opening your account, go to the developer's section and access your API keys.
In the developer's section, you will find two keys: publishable key y secret key; copy them in your .env file.
frontend/.env
Stripe dashboard
2. Integrate Stripe into your Vue 2 App.
- Once you have opened your account with Stripe, copy the script tag into the head of index.html.
frontend/public/index.html
-
Immediately after getting the subtotal from the customer, create the onclick event to call the server and request the payment intent.
Use the
paymentIntent()
function in different parts of your app, place it in the login form and the guest form.
frontend/src/views/Cart.vue
frontend/src/views/Cart.vue
To send the payment intent from Vue to the server, we are going to use Vuex and Axios. Vuex is the state management for Vuejs applications, and Axios is the http requests manager. In the following code, retrieve the user object and the shopping cart. Send the shopping cart and calculate the transaction total based on the product id. After sending these objects, get the response from the server with the client secret and the tax calculation, send them to the mutations and store them in the state.
frontend/src/store/index.js
frontend/src/store/index.js
- Initialize the state with the client secret in null and tax in 0.
frontend/src/store/index.js
3. Mount the Stripe Card Element.
Once we have the client secret store in the state, we can use it to mount the Stripe card element. This card element will show to the customer the Stripe form in which the customer will insert his credit card number. Remember Stripe has the transaction total. With this card element, the customer is authorizing and finalizing the transaction.
frontend/src/views/Checkout.vue
Inside the script tag of Vue, import the store from vuex so we can access the client secret.
Use your public secret key and window.Stripe()
function to get the form element from Stripe.
Your public secret key must be stored in the .env file; never put it directly in the code! Once we get the payment element,
we can modify its appearance Stripe appearance-api.
frontend/src/views/Checkout.vue
- Inside export default, mount the Stripe Element using Vue's mounted function.
frontend/src/views/Checkout.vue
-
The
DisplayError()
function will show the customer if his card has errors such as insufficient funds.
frontend/src/views/Checkout.vue
Finally, send the transaction through the Submit function. It is important to note that /thankyou/
is the URL parameter of our last route.
This is located in the directory under Thankyou.vue
. this project uses Vue Router for easy moving between pages.
Import mapState
from Vuex to extract variables from the state and send them with the address object to Stripe.
frontend/src/views/Checkout.vue
4. Extracting Properties from Stripe.
If you need to retrieve customer information from Stripe, you should do so at the Thankyou.vue
page.
Through the javascript function URLSearchParams()
, we retrieve the payment_intent_client_secret
from the URL,
this will be used by the Stripe retrievePaymentIntent()
function to access the transaction object and be able to send the properties to our server.
Once the values have been sent, retrieve the response, and if everything is ok, use COMMIT_CHECKOUT
and CLIENT_SECRET
environment variables to clear the state.
frontend/src/views/Thankyou.vue
frontend/src/store/index.js
- Clear the state on successful checkout. For the server-side code of this Stripe implementation checkout this blog: How to Connect Stripe to Django Rest Framework? .
frontend/src/views/Thankyou.vue