How to Unit Test your Express Js API With Jest?
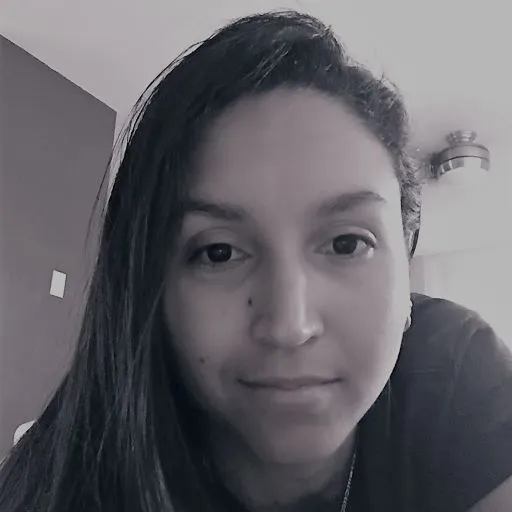
By:
Denisse Abreu
December 11, 2022 8pm ET
Eng/Spa 5-min
Hello 👋🏼, This is the third part of my Unit Testing Tutorial.
In this occasion, we'll learn how to test an Express Js API.
The goal here is to test the request and responses of our API and
ensure that it runs smoothly.
Our working environment is Express Js, Axios, Express Validator, and Jest for Unit Testing.
Let's start!
Set Up your Express Js Server.
- We'll start by creating an empty folder and initializing our project there.
Git Bash
mkdir express-tutorial
cd express-tutorial
npm init -y
- Once the project has been initialized, install the Express Js library and the Express Validator.
Git Bash
npm i express express-validator
Go to the project root, create a routes
folder, and inside create routes.js
; this
file will hold all our routes. Learn more about the
express.Router()
Git Bash
mkdir routes
cd routes
touch routes.js
- Open this file in your favorite editor and copy the following code.
routes/routes.js
-
Finally, create
index.js
in the project root and copy the following code.
index.js
Run your first API test.
Let's get ready to run our first test; install Jest, Axios, and the Nodemon library; all in development mode. Soon, Node will be able to watch for file changes, so in the future, you will not need to install Nodemon.
Git Bash
npm i -D jest nodemon axios
Add the scripts to package.json
, so we
don't have to type the commands constantly. If you want the test to
restart on every file change, add --watchAll
to Jest.
package.json
-
Create a
__test__
folder in the project root, and inside createindex.test.js
.
Git Bash
mkdir __test__
cd __test__
touch index.test.js
__test__/index.test.js
Run your server with npm run dev
, then
on a separate terminal, run the test with npm test
;
make sure that the status code return from the server is 200 and the data is the
Hello World! string.
Test a simple Login route.
Let's build a simple login route to test a POST http request.
First, add the following code to routes.js
routes/routes.js
-
Go to
index.test.js
and test your login route.
__test__/index.test.js
How to Test the Express Validator?
In the last part of this testing tutorial, we'll use the
Express Validator
to test the login route. First, go to routes.js
and modify
the login route. Return status code 400 if the validation fails.
routes/routes.js
Go to index.test.js
and test the
Express Validator. When testing APIs, don't forget to check
error codes and messages. See you next time!
__test__/index.test.js