How to Unit Test your React TypeScript App With Vitest and React Testing Library.
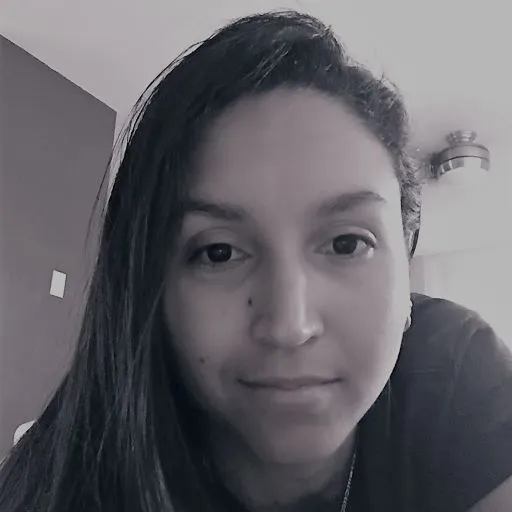
By:
Denisse Abreu
November 27, 2022 3pm ET
Eng/Spa 8-min
Hello 👋🏼, This is the second part of my Unit Testing Tutorial.
We'll learn how to test a React TypeScript application.
Our working environment is Vite, TypeScript, Vitest for Unit Testing, and the React Testing Library.
First, we'll make some simple test cases; Then, we'll progress into more advanced topics, like how to test React Router Dom and Test a React Context.
Let's start!
Set Up your React App with Vite and Vitest.
- Install Vite and choose React TypeScript as your template.
Terminal
npm create vite@latest testing-tutorial -- --template react-ts
cd testing-tutorial
npm install
npm run dev
After checking that your app works, install Vitest, happy-dom as its testing environment, and the React Testing Library all as dev dependencies; Check the Vitest installation for more info.
Terminal
npm install -D vitest happy-dom @testing-library/react
-
Go to
vite.config.ts
and add the test object with its type definition.
vite.config.ts
-
Add the test script to
package.json
package.json
Run your first Vitest Case.
To run the first test, create a new file in the root
and call it App.test.tsx
. This file will contain
all the tests related to the App.tsx
file.
In the following code, mount the App component and test that it exists
with its elements.
src/App.test.tsx
Terminal
npm install -D @testing-library/jest-dom
-
Go to the
src
folder, and create thesetupTest.ts
; This file will import the Testing Jest Dom Library globally.
src/setupTest.ts
-
Go to
vite.config.ts
and add the newly created file to the test object.
vite.config.ts
-
Lastly, go to
tsconfig.json
and include the path of thesetupTest.ts
.
tsconfig.json
- Now you can use additional testing functions using the Testing Library Jest Dom 👍.
src/App.test.tsx
Testing React with the User Event Library.
Next, we'll test user events, like buttons, links, and logos. First, we'll use the counter that came with the Vite default installation, get the Button by text using the React Testing Library, and check that the value increments.
src/App.test.tsx
Example test using the Testing Library User Event. First, install the library. In this test case, we check that the logo receives one click.
Terminal
npm install -D @testing-library/user-event
src/App.test.tsx
How to test the React Router?
We'll start by installing the React Router Dom. and create an about link with its about page.
Terminal
npm install react-router-dom
-
First, create a new
routes
folder; Inside, createabout.tsx
and insert the following code.
Git Bash
cd src
mkdir routes
cd routes
touch about.tsx
src/routes/about.tsx
-
Go to
main.tsx
add the Browser Router and import the about route.
src/main.tsx
-
Add your link to the
App.tsx
and test that it works.
src/App.tsx
- Create a test case to check the about link receives one click from the user.
src/App.test.tsx
How to test a React form input?
In this part of the tutorial, we'll create a reusable input field and test it
with its props. First, create a components
folder in the root;
then, build form-input.tsx
and copy the following code.
Remember! to use TypeScript and pass the label in an actual project.
Git Bash
cd src
mkdir components
cd components
touch form-input.tsx
src/components/form-input.tsx
-
Create the
form.tsx
in thecomponents
folder.
src/components/form.tsx
-
Now that we have a form, it's time to test it. Create a
test
folder inside thecomponents
, then createform.test.tsx
.
Git Bash
cd src && cd components
mkdir test
cd test
touch form.test.tsx
src/components/test/form.test.tsx
How to Create and Test the React Context?
Let's create a simple React Context that will receive the email input we previously created, then test it. This lesson will have major refactoring as adding a context will change how the App works.
-
First, create the
context
folder, then make theuser-context.tsx
, this file will store all the states related to the user.
Git Bash
cd src
mkdir context
cd context
touch user-context.tsx
src/context/user-context.tsx
-
Go to
main.ts
and wrap the App component inside the user provider.
src/main.tsx
-
Go to the
form.tsx
and bring the Context; so it can store the email value.
src/main.tsx
-
Add a new test to the
form.test.txs
to check if the user context is passing values, and displaying the email in the Dom. That's it for now, see you next time!
src/components/test/form.test.tsx