Unit test your Vue 3 App with Vitest, TypeScript, and Vue Testing Utils.
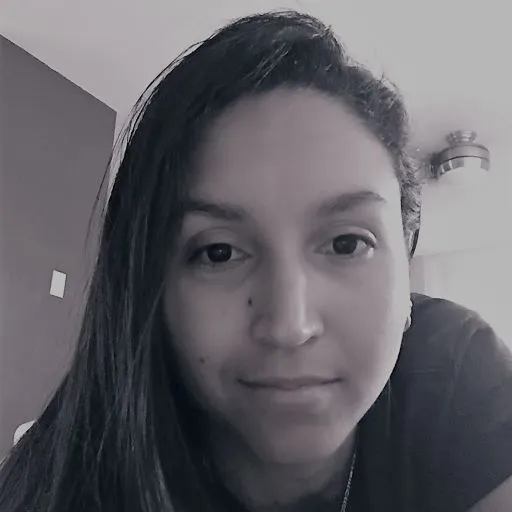
By:
Denisse Abreu
Nov. 6, 2022 7pm ET
Eng/Spa 10-min
Hello ๐๐ผ, Let's talk about testing. Testing is one of those subjects that, for whatever reason, is left out of most tutorials. Unit Testing is vital
for the performance and operation of your application; it will ensure your App runs smoothly through its lifetime. Remember, as time passes,
we update and change our App features, which can have breaking changes that only will be detected by running Tests.
In this first part of the Testing tutorial, we'll work with Vue 3, Vitest, and Vue Testing Utils.
We'll install a Vue 3 project and build a simple form component.
Let's get started!
First, install a Vue 3 project. If you don't know how to install Vue 3,
follow Vue 3 installation guide.
Open your terminal and run: npm init vue@latest
.
TerminalProject name: testing-tutorial Add TypeScript? Yes Add JSX Support? No Add Vue Router for Single Page Application development? Yes Add Pinia for state management? Yes Add Vitest for Unit testing? Yes
- After the project installation, go to the root of the testing-tutorial, install the libraries and run the project in development mode.
Terminalcd testing-tutorial npm install npm run dev
Run your First Unit Test.
Open the terminal and run your first unit test. You can find the command to run this test in the script part of the package.json
manifest; It consists of a simple test to check if the HelloWorld.vue
component mounts and pass a message prop.
The test should pass.
Terminalnpm run test:unit
Test the front-page components by creating TheWelcome.spec.ts
inside the __tests__
folder. In the following test,
check that the component renders with an SVG and an anchor tag; Also, check that the anchor tag clicks.
src/components/__tests__/TheWelcome.spec.ts
How to test a Vue 3 form component?
We'll start the lesson by testing a simple form component.
Go to the components
folder,
and create a form with one input element and a button.
Import this component in the HelloWorld.vue
file.
src/components/TheForm.vue
-
Go to
HelloWorld.spec.ts
and add more test cases.
src/components/__tests__/HelloWorld.spec.ts
How to test The Vue Router?
Before testing the Vue Router, We'll do some refactoring.
Go to the components
folder, and create
a Header.vue
file. Move the header
section of the App.vue
into the newly created
file. Your App.vue should look like the following code.
src/App.vue
src/components/Header.vue
Now that we have a good separation of concerns, start the Router Testing.
In the __tests__
folder, create a Header.spec.ts
and fill it with new test cases.
Also, I added type="button"
and id="link"
to show you how to grab these elements.
src/components/__tests__/Header.spec.ts
How to test Pinia?
In this part of the tutorial, we'll use Pinia, the state manager of Vue applications. We'll mock some values, send them to the store, and check that the values exists in the state. But first, let's test the counter store that Pinia installed by default.
Go to the stores
folder and create __tests__
.
Inside that folder create, counter.spec.ts
, and
fill it with the following code. Remember to run your test!
src/stores/__tests__/counter.spec.ts
src/stores/counter.ts
In the last part of this tutorial, we'll complicate the store and create a user store,
where we insert some values and check that they are in the store.
Go to the stores
folder, and create user.ts
.
src/stores/user.ts
-
Create
user.spec.ts
in the__tests__
folder and run your test.
src/stores/user.spec.ts