How to create an Auth system with Firebase and React?
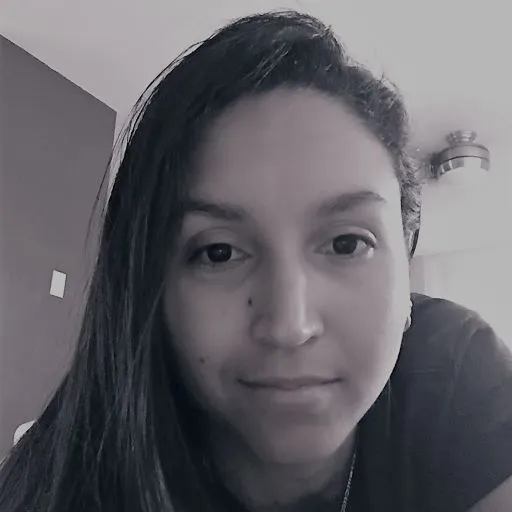
By:
Denisse Abreu
January 15, 2023 7pm ET
Eng/Spa 6-min
Hello 👋🏼, I decided to start this year with Firebase! We'll start this tutorial
by creating a simple login form with React and TypeScript and then connecting it to the Firebase authentication service using email and password.
For this project, you need a Firebase Web App; if you don't have a firebase project, follow their
add Firebase to your project instructions.
The second part of this tutorial is How to reset a password in the Firebase Auth System?
in case you want to review it first.
Our working environment is Vite, React Router Dom, and React Context.
Let's start!
Firebase Authentication Demo
Initialize Firebase Authentication in the console.
After creating a Firebase Project, provision the authentication service by going to the Build tab in the Firebase console's left sidebar, then enable sign-in with email and password, and add a test user.
Firebase Dashboard Image
Firebase Auth Users Image
Set Up React TypeScript with Vite.
- Install Vite and choose React TypeScript as your template.
Terminal
npm create vite@latest auth-tutorial -- --template react-ts
cd auth-tutorial
npm install
npm run dev
After checking that your app works, install React Router Dom and the Firebase tools; check the React Router Dom tutorial for more info.
Terminal
npm install react-router-dom firebase
Create the Firebase Configuration Folder.
Before getting into React, we need to set our Firebase configuration
files. Create a firebase
folder, and
inside create two files: firebase-config.ts
and firebase.ts
Git Bash
cd src
mkdir firebase
cd firebase
touch firebase-config.ts && touch firebase.ts
-
Copy the following code into your
firebase-config.ts
, and replace the variables with your own.
src/firebase/firebase-config.ts
- There's no need to type the config variables; as you can see, TypeScript will auto-infer them.
Firebase configuration object
-
Bring the
firebase-config.ts
to the Firebase file, so you can start making calls to Firebase.
src/firebase/firebase.ts
Set Up the React Authentication Context.
When building an authentication system in React or any Javascript application, it is crucial to use storage to keep track of the user's state. In this tutorial, I'm using the Context because it's lightweight and doesn't require too much setup; If you're building a complex application, use Redux instead.
First, create a context
folder and auth-context.tsx
;
then initialize the current user state as null. When the user clicks the sign-out button,
redirect him to the home page.
Git Bash
cd src
mkdir context
cd context
touch auth-context.tsx
src/context/auth-context.tsx
Create the Routes for your Auth System.
In the last part of this tutorial, we'll work on the routing and the login UI.
First, go to main.ts
and bring the Context and the
BrowserRouter
from React Router Dom.
src/main.tsx
- Create a simple Home page and Profile page under the routes folder.
Git Bash
cd src
mkdir routes
cd routes
touch home.tsx && touch profile.tsx
src/routes/home.tsx
src/routes/profile.tsx
-
Bring the two routes to
App.tsx
.
src/App.tsx
In the example above, we used currentUser
to stop people from
accessing the profile page through the URL. Another way to do this
is by using a Higher Order Component (HOC) that will wrap the profile
route and check if there is an active user before letting people into
the profile page.
- Create a components folder, and inside, create require-auth.
Git Bash
cd src
mkdir components
cd components
touch require-auth.tsx
src/components/require-auth.tsx
Refactor App.tsx
to use the HOC.
src/App.tsx
This HOC is reusable and will give you more flexibility on what routes you want to protect from malicious users. This HOC also saves the router history, redirecting the user to the page he wanted to go to after a successful login; for more info about the auth router, go to Remix Github. Visit How to reset a password in the Firebase Auth System? if you want to integrate the Firebase password reset into this tutorial.