How to reset a password in the Firebase Auth System?
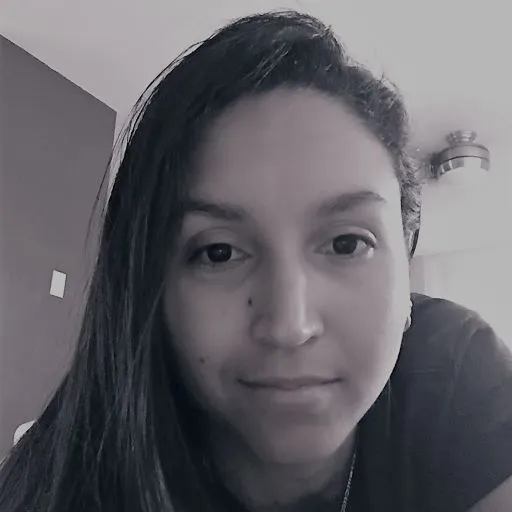
By:
Denisse Abreu
February 01, 2023 9pm ET
Eng/Spa 8-min
Hello 👋🏼, In the last tutorial, we learned how to create an Authentication system with Firebase and
React
using email and password. In this tutorial, we'll learn how to add the password reset,
commonly known as forgot password link, and how to initialize the Firebase Auth Emulator.
The Firebase Emulator Suite is a development environment that Firebase allows web developers to use for
testing and prototyping, so we don't have to mess with the production database, auth, and hosting resources.
Consult the
Firebase Emulator Suite for more info.
Let's start!
Reset Password Flow Chart
Connect the Firebase Emulator Suite to React.
Go to the firebase.ts
and instruct your App to connect to the Emulator Suite when running
in a development environment. Bring two new functions from Firebase:
sendPasswordResetEmail()
and confirmPasswordReset()
.
These functions are void promises, meaning they will not return anything other than the error code from Firebase;
create your own messaging system and catch the error.
src/firebase/firebase.ts
Create the forgot password and the Password Reset Forms.
-
Go to the routes folder and create two new routes:
passwordReset.tsx
andforgotPassword.tsx
.
Git Bash
cd src
cd routes
touch passwordReset.tsx && touch forgotPassword.tsx
First, we'll work on the forgot password form. This form consists of a simple HTML input element that will send the user email to Firebase and check if it exists in the Auth system. If the email doesn't exist, your App will receive a user not found error.
src/routes/forgotPassword.tsx
The following code belongs to the password reset file. This form is
crucial and needs to be tested thoroughly, the user will redirect
himself back to the App through the link sent by Firebase to his
email inbox. This link will have a unique code called
oobCode
; Extract this code from the URL
and send it back to Firebase through the confirmPasswordReset()
function. Firebase will match this code and allow the password reset if it's the same.
src/routes/passwordReset.tsx
- Add the forgot password link to the login form.
src/routes/home.tsx
-
Lastly, bring the new routes to the
App.tsx
. The path to the password reset form will point to the emulator for now.
src/App.tsx
Initialize the Auth Emulator Suite.
Before running the Emulator, you will need a Firebase account and a project;
you can upload this project or use a demo or testing project;
with the auth system already provisioned. Open your terminal within the root folder,
and type firebase login
; after logging in,
run firebase init emulators
.
The CLI will ask how you want to set up your project;
for this tutorial, we need the Authentication Emulator, port 9099, and the emulator UI.
When your Firebase project is ready, run in the terminal firebase emulators:start
and visit http://localhost:4000/
. Consult the
Firebase CLI reference and
Connect your App to the Authentication Emulator
if you still need help with the setup.
Firebase Emulators CLI
- Add a test user to the Firebase Auth Emulator.
Adding User to the Auth Emulator
Return to the Application, open a new terminal, and run the project. You will notice that Firebase has added a warning sign to differentiate the Emulator from the production project, "Running in emulator mode. Do not use with production credentials."
Login Form Emulator
Start to test the auth system; when you reach the "check your inbox" message, go to the console, grab the link outputted by the emulator, and paste it into your web browser's address bar; hit enter, and you should see the reset password form.
Reset link Firebase Emulator CLI
After changing your password, return to the Emulator, hit refresh, and check that the password
has been changed. You will notice the user will be verified this time. Continue to manually
test the reset password link by deleting the oobCode
, changing it
for a different code, and reusing the same URL link repeatedly, be creative here and think like a hacker!
How the Firebase Reset Password works in Production.
When you finish testing the auth system and are ready to deploy your project,
go to the Firebase Dashboard, Authentication, templates, and password reset.
Click edit template and go to the bottom to Customize Action URL.
There, set the path to your user action landing page; Don't include the parameters mode
and oobCode
; they will be appended by Firebase dynamically.
If you don't know how to create a user action landing page and a higher order
component to redirect the user depending on the action the user wants to achieve (reset the password),
check:
Create a landing page for the Firebase User Actions
See you next time!
Customize Action URL Firebase
src/App.tsx