How to Register users with Firebase and React?
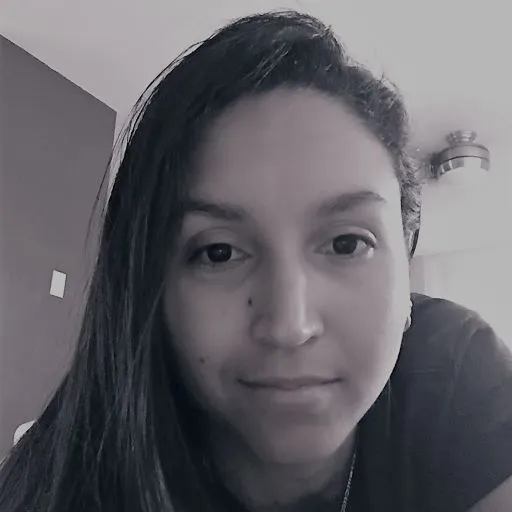
By:
Denisse Abreu
March 25, 2023 4pm ET
Eng/Spa 4-min
Hi 👋🏼, in this tutorial, we'll learn how to register users with the
Firebase Authentication System and React. If you want to check how to log in
users and set up the tutorial from the start, visit
How to create an Auth system with Firebase and React?.
Our working environment is Vite, React with TypeScript, and the Firebase npm package.
Let's start!
Set Up the Firebase Create User Functions.
In the firebase.ts
file that we previously created,
add the createUserWithEmailAndPassword()
function.
The minimal information to open an account using the Firebase auth is the email and
password; you can also collect the user name outputted as a displayName
, the phone number, and
a photo hosted somewhere on the internet.
In the following code, create the user, send the email verification to the user's inbox, and update his profile with the username and an example image from robohash.org.
src/firebase/firebase.ts
The following code demonstrates how to collect the email verification code
we send to the user's inbox. Import the applyActionCode()
from Firebase and extract the oobCode
from the URL.
After the user has registered successfully, he will be login by Firebase
automatically regardless of his email verification status.
src/firebase/firebase.ts
Create the routes and components for the Firebase email verification.
-
Create the
register.tsx
andconfirmEmail.tsx
routes.
Git Bash
cd src
cd routes
touch register.tsx && touch confirmEmail.tsx
- Collect the user information and remember to validate the inputs according to your application needs.
src/routes/register.tsx
Extract the oobCode
from the URL, and send it
to the Firebase file through the useEffect()
hook.
Delete the React StrictMode if running twice in development, or use a cleanup
function to clear the oobCode
after running it for the first
time. You can learn more about the
cleanup function in the React docs.
src/routes/confirmEmail.tsx
Create a landing page for the Firebase User Actions.
While creating this tutorial, I notice that Firebase only allows one URL for all user actions like email verification, password reset, etc. In order to redirect the user to the correct action (email verification), we need to build a higher-order component.
-
Create a landing page for the user actions. Go to routes and add
authUserActions.tsx
.
src/routes/authUserActions.tsx
Go to the components folder, create the auth-actions.tsx
,
and get the mode from the URL and the oobCode.
Navigate to different routes depending on the URL mode. Remember, in this tutorial,
we're focusing on email verification.
src/components/auth-actions.tsx
-
Now that we have all the components and routes, add them to the
App.tsx
file.
src/App.tsx
To finish this tutorial, go to the Firebase console, authentication, and
customize your action URL template to point to our user actions landing page.
Remember! You can always test this registration process using the
Firebase Emulators,
change the path to emulator/action
, and you're
good to go. See you next Time!
Customize Action URL Firebase