How to make a Star Rating System With Django Rest Framework?
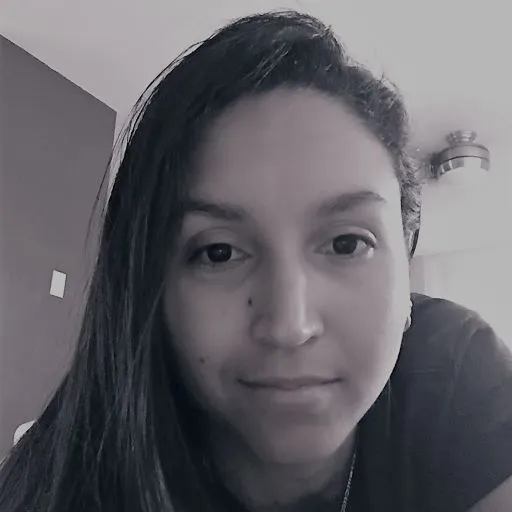
By:
Denisse Abreu
Mar 23, 2022 8pm ET
Eng/Spa 2-min
Hello 👋🏼, In this article, I will teach you how to make a star rating system for your online shopping store. If you want to review the client-side first, do it here: How to build a star rating system with Vue 2, Vuetify, and Django? First, let's set up our environment. For our backend server, I'm using Django and Django Rest Framework. For the client-side, I'm using Vuejs Options API, and for the star rating UI/UX, I'm using the Vuetify star rating component. This guide will assume that you have previous experience dealing with Django. I already have the project in my repository; you can fork my Django Shopping Store. Let's start.
- Install all the necessary libraries.
Install through pippip install djangorestframework
Let's start by creating our model. First, bring the Product model and link each product to its star rating system database; build the database using words as table headers. The table data is a positive integer that will increase one by one. The string dunder method will evaluate the database and return the column with the large number.
store/modelsclass Rating(models.Model): product = models.ForeignKey(Product, related_name='rating', on_delete=models.CASCADE) one = models.PositiveIntegerField(default=0, null=True, blank=True) two = models.PositiveIntegerField(default=0, null=True, blank=True) three = models.PositiveIntegerField(default=0, null=True, blank=True) four = models.PositiveIntegerField(default=0, null=True, blank=True) five = models.PositiveIntegerField(default=0, null=True, blank=True) class Meta: ordering = ['product'] def __str__(self): # Extract all rating values and return max key. # Reverse this Dict if there is a tie and you want the last key. rating_list = { '1': self.one, '2': self.two, '3': self.three, '4': self.four, '5': self.five } return str(max(rating_list, key=rating_list.get))
Django Admin Dashboard
Create a serializers.py
file inside the store folder. Bring the Product model with all its fields and append the rating list at the end.
store/serializersfrom .models import Product from rest_framework import serializers class ProductSerializer(serializers.ModelSerializer): rating = serializers.StringRelatedField(many=True) class Meta: model= Product fields = ['id', 'name', 'slug', 'image', 'price', 'qty', 'inventory', 'description', 'rating']
We are now ready to implement our class-based view; import the viewsets
from the Rest Framework, ProductSerializer
from
the serializers file, and the product model.
store/viewsfrom rest_framework import viewsets from .serializers import ProductSerializer from .models import Product # Get all products in random order class ProductViewSet(viewsets.ModelViewSet): queryset = Product.objects.all().order_by('?') serializer_class = ProductSerializer
Last but not least, we must implement our routes so the server can receive the product's object
with its rating when the front end sends it.
Create the routes in store URLs file, import ProductViewSet
from the views file,
and routers from the Django Rest Framework.
The router variable from the Rest Framework works as a route controller.
store/urlsfrom django.urls import path, include from rest_framework import routers from .views import ProductViewSet router = routers.DefaultRouter() router.register(r'products', ProductViewSet) urlpatterns = [ path('', include(router.urls)), path('api/', include('rest_framework.urls', namespace='rest_framework')) ]
The next image shows the product object that will be sent to the front-end, the rating list means that the red cup has 3 stars. If you want to implement the client-side of this guide, do it here: How to build a star rating system with Vue 2, Vuetify, and Django?
Rest Framework API Object