How to build a Star Rating System with Vue 2, Vuetify, and Django?
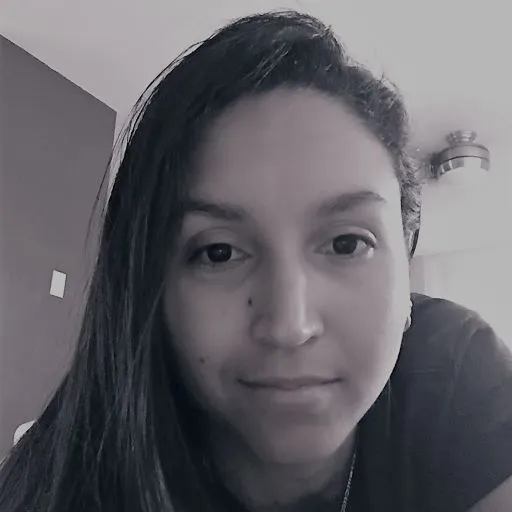
By:
Denisse Abreu
Mar 29, 2022 6pm ET
Eng/Spa 6-min
Hi 👋, this is the second part of our guide: How to make a Star Rating System with Django Rest Framework? if you are reading this article for the first time, our working environment is Vue 2, Vuetify, and Django Rest Framework. If you don't have a project, you can copy mine here: Django Shopping Store
In the first part of this guide, we sent the product object to the frontend;
the following code shows how we can get it using the getProducts()
function in the actions part of Vuex.
frontend/src/store/index.js
Mutate the data that we received from
Django Rest Framework
by committing the SET_PRODUCTS
environment variable with its payload.
frontend/src/store/index.js
- After the mutation of the product objects, the data is ready to be stored in the state.
frontend/src/store/index.js
1. Build The Star Rating Component.
Now it's time to build the
Vuetify
star rating component. This component will receive two props,
the itemId
and the itemRating
.
After getting these props, we need to mutate the itemRating
so the template can use it in its v-model value.
Use the data()
function to mutate the itemRating
and convert it into an integer.
Use the @input="changeVal()
to commit the rating and the item id to Vuex.
frontend/src/components/StarRating.vue
frontend/src/components/StarRating.vue
- Import the star rating component and place it inside the product object, it needs access to the product id and rating.
frontend/src/views/Home.vue
2. Star Rating Storage Management.
Now it's time to retrieve the star rating object sent by the star rating component.
In the Vuex mutations, destruct the payload and use the findIndex()
javascript function to search the stars rating array in the state.
This function will match the item id in the starRating
payload to the item id
in the state stars array; if the itemId
doesn't exist in the state stars array,
a new object will be created using the push()
function; otherwise, the state stars array will be
assigned the rating value from the payload.
frontend/src/store/index.js s
Vue js Devtools
3. Sending the Star Rating Object to the Backend.
After the user rates your product, you need to think about how you want to send the star rating object to the server. One option is to send it when the customer logs into the application; you can make an open system where everybody can rate your products or send the star ratings when the customer completes the checkout process.
frontend/src/store/index.js
- Receive the order object through this URL in the Django Rest Framework.
mystore/orders/urls.pyfrom django.urls import path, include from rest_framework import routers from .views import OrderViewSet router = routers.DefaultRouter() router.register(r'order', OrderViewSet) urlpatterns = [ path('', include(router.urls)), path('api-post/', include('rest_framework.urls', namespace='order')), ]
In the last part of the Star Rating System, start to loop inside the rating request.
Get the item id and the rating value; Bring the rating model, match the product
with the itemId
from the request, and increase the quantity by one.
Check my repository for the complete order view:
Django Shopping Store
mystore/orders/views.pyclass OrderViewSet(viewsets.ModelViewSet): queryset = Order.objects.all().order_by('-created_at') serializer_class = OrderSerializers def create(self, request): if request.method == 'POST': rating = request.data.get('order')['rating'] if rating: for i in rating: item_id = i.get('itemId') value = i.get('value') r = Rating.objects.get(product=item_id) if value == 1: r.one += 1 elif value == 2: r.two += 1 elif value == 3: r.three += 1 elif value == 4: r.four += 1 elif value == 5: r.five += 1 r.save() serializer = self.serializer_class( data=order, context={'request': request} ) if serializer.is_valid(): serializer.save() return Response(status=status.HTTP_200_OK) return Response( serializer.errors, status=status.HTTP_400_BAD_REQUEST ) return Response(status=status.HTTP_405_METHOD_NOT_ALLOWED)